31 August 2010 - 13:00There’s Always Another Release
As Atul mentioned in “The Social Constraints of Bettering The Web” [toolness.com], Account Manager will likely not make its way into Firefox 4. He points out one of the biggest bottlenecks as getting approval from Firefox product drivers:
Within Mozilla, I see my coworkers vie for the attention of this tiny handful of gatekeepers. People in charge need convincing; the clever social engineer has a lot of power when it comes to navigating this landscape.
This last step of getting approval comes at the very end of a long line of work. While Dan and I have been busy implementing the core feature and making sure it doesn’t regress performance and tests, a good number of people have been involved both inside and outside of Mozilla to design the user interface, to flesh-out the spec and to integrate the feature into sites or as plug-ins.
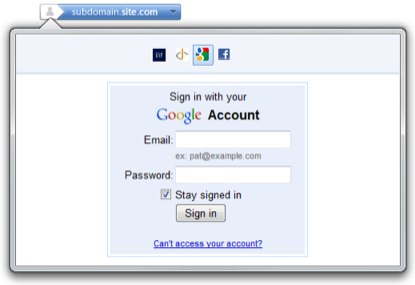
Alex Faaborg's mockup of letting users pick different types of accounts
But this approval process affects people outside of Mozilla as well. New developers in the community hear about neat features of the upcoming Firefox and want to help by hacking on patches of related features. For example, the status bar removal in Firefox 4 has a number of side-effects including the removal of the download statusbar. Alex Limi has suggested ways for “Improving download behaviors in web browsers” [limi.net], which would add a new toolbar interface, and while there are initial patches from multiple community members, it seems unlikely to make it to Firefox 4.
I even ran into this same roadblock a couple years ago when I was trying to get the AwesomeBar into Firefox 3. Back then I was a random community member that had a good idea and was able to hack on stuff in my “free” (ha! ;)) time. It was only after a lot of prodding and persistence that got just a bit of what I worked on into Firefox 3, but that was all very stressful as I looked back on “Why I Worked On Firefox.”
But fear not community members! There’s always another release. The product drivers are not approving patches during this beta-crunch time because there’s always some risk involved with changes (especially those “it’s just a one line change” fixes :D). New changes typically are followed up by a number of new issues and patches that then need to be additionally hacked on, tested, reviewed, and approved. So just because it doesn’t get approval now doesn’t mean it won’t be accepted when the tree is more open.
Additionally, patches usually come with a number of dependent fixes that might be able to land first. And in the case of Account Manager, I’ve already gotten in some changes into Firefox 4 that improve the new PopupNotifications (used by Geolocation and Add-ons) and testing infrastructure. Some other useful changes that could land independently of Account Manager are some upgrades to the Password Manager and networking APIs. So while the core feature might not be in yet, the platform is made better and ready for it.
So keep hacking away and perhaps your feature will be ready to land on the open tree after Firefox 4 branches. And then it’ll have many months to bake and get tested by other community members and eventually be seen by millions of Firefox users. 🙂
10 Comments | Tags: Account Manager, AwesomeBar, Development, Mozilla